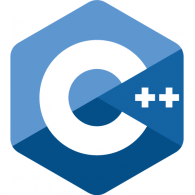
Write a C++ program , that prints all the Fibonacci numbers , which are smaller than or equal to a number k(k≥2) ,which was entered by the user.
- The Fibonacci numbers are a sequence of numbers,where then-th number of Fibonacci is defined as:
- Fib(0)=0,
- Fib(1)=1,
- Fib(n)=Fib(n-1)+Fib(n-2) Usage :> Input of k:21 > Fibonacci numbers<=21:01123581321
Solution 1 –
The solution below will answer following questions :
1. Write a program to generate the Fibonacci series in C++.
2. Fibonacci series in C++ using for loop.
3. How to print Fibonacci series in C++.
4. Write a program to print Fibonacci series inC++.
5. How to find Fibonacci series in C++ programming.
6. Basic C++ programs Fibonacci series.
#include<iostream> using namespace std; int main (void) { int r=0,a=0,n,b=1,i,c; char redo; do { a=0; b=1; cout<<"enter the the value of n:"; cin>> n; if(n>100){ cout<<"enter some lessor value"<<endl; } else{ cout<<a<<endl; for (i=0;i<=n;i++) { c=a+b; a=b; b=c; cout<<"serial no."<<i+1<<"=="; cout<<a <<endl; } cout<<"enter y or Y to continue:"; cin>>redo; cout<<endl<<endl; } } while(redo=='y'||redo=='Y'); return 0; }
Solution 2 –
If we want to Write a program to generate the Fibonacci series in C++ using recursion, the follow the solution below :
/** Fibonacci series in C++ using recursion by codebind.com */ #include <iostream> int Fibonacci(int x) { if (x < 2){ return x; } return (Fibonacci (x - 1) + Fibonacci (x - 2)); } int main(void) { int number; std::cout << "Please enter a positive integer: "; std::cin >> number; if (number < 0) std::cout << "That is not a positive integer.\n"; else std::cout << number << " Fibonacci is: " << Fibonacci(number) << std::endl; return 0; }
Solution 3 –
The solution below will answer following questions :
1. Fibonacci series using array in C++
2. Fibonacci series program in C++ language
3. Source code of Fibonacci series inC++
4.Write a program to print Fibonacci series in C++
/** Fibonacci series in C++ using array by codebind.com */ #include <iostream> int main(void) { int i,number; long int arr[40]; std::cout << "Enter the number : "<< std::endl; std::cin >> number; arr[0]=0; arr[1]=1; for(i = 2; i< number ; i++){ arr[i] = arr[i-1] + arr[i-2]; } std::cout << "Fibonacci series is: "; for(i=0; i< number; i++) std::cout << arr[i] << std::endl; return 0; }
- C++ Simple Programs And Examples
- C++ – Hello World Program
- C++ – Simple calculator
- C++ – Even and Odd
- C++ – Swap two numbers
- C++ – Prime Number
- C++ – Find Perfect Number
- C++ – Factorial of Number
- C++ – Fibonacci Series
- C++ – Human Resource Management Program
- C++ – Calculate number of characters, words, sentences
- C++ – Subtract two Strings
- C++ – Processing of the Students structure
- C++ – Program with Matrices
- C++ – Calculate Equation
- C++ – Arrange Numbers in Ascending order
- C++ – Check Armstrong Number
- C++ – HCF and LCM
- C++ – Square Root of a Number
- C++ – Cube Root of a Number
- C++ – ASCII Code for Characters and numbers
- C++ – Check Palindrome Number
- C++ – Bubble sort
- C++ – Random Number Generator
- C++ – Sum of ODD Numbers in the Given Range
- C++ – Display current date and time
- Formula Based Programs
- C++ – Leap Year
- C++ – Surface Area and volume of cone
- C++ – Solve Quadratic equation
- String Based Programs
- C++ – String into Upper case or lower case
- C++ – Concatenate Strings
- Array Based Programs
- C++ – Program with Matrices
- Print Any Patterns
- C++ – Print 5 rows of 10 Stars (*)
- C++ – Half Pyramid of Stars (*)
- C++ – Half Pyramid of numbers
- C++ – Print Triangle of Stars
- C++ – Display Reverse pyramid.
- C++ – Print Alphabet Pattern
- C++ – Diamond of Star
- C++ – Pascal Triangle
- C++ – Floyd Triangle
- C++ Conversion
- C++ – Convert decimal to Hexadecimal
- C++ – Decimal to Binary
- C++ Sorting algorithms & Techniques
- C++ – Bubble Sort
- C++ – Insertion Sort
- C++ – Selection Sort
- C++ – Merge Sort
- C++ – Quick Sort
- C++ Handling Files
- C++ – How to get Current Directory in Linux/Windows
- C++ – How Create a Text File and Write in It
adding comments in the program would be very helpful