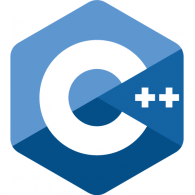
Mini-Project: Human Resource Management Program
Consider that you want to write a program for the company called XYZ which produces medical instruments. This company is divided into 2 departments: Manufacturing Department (MD) and Human Resource Management department (HRM). You are requested to write a program for HRM department of company XYZ including the maximum number of 100 employees. shows main menu for your program. You are allowed to re-design this menu but with the same functionalities. Note that after each step you should come back to main menu.
Welcome to Human Resource Management (HRM) Software of Company XYZ. To do specific task please choose one of the following commands.
1. Add new employee
2. Delete employee information
3. Update employee information
4. Make reports based on specific field
5. Search employee
6. Quit
Please enter the related number of your requested command?
The field “PersonalID” of class Person should be unique and auto-increment with the initial value of 8921001 for the first employee. It means that user should not enter the value for “personalID”.
Class HRM should include following private fields:
Array of employees with the type Person (Private)
The actual amount of staff which is stored in Array of employees(private)
Class HRM should include following public member functions:
AddPerson()
DeletePerson(…)
UpdatePerson(…)
ReportList(…)
SearchPerson(…)
Please enter the first name of an employee? Alex
Please enter the last name of an employee? Peterson
How many hours a week is his/her work? 35
How much per hour is his/her salary? 20
The employee with the following information has been added to the system:
First Name Last Name Personal ID Salary per year (Euros)
————– ————– ————– —————————
Alex Peterson 8921001 36400
Do you want to add another employee (y/n)? n
2. DeletePerson(…)
This function allows user to delete the staff information. Since the only unique value in the list of employees is “Personal Number”, therefore delete function should be based on this field. Note that in order to enter the correct personal number of employee you need to find his/her personal ID using the function SearchPerson(…).
Please enter the personal number of an employee? 2425374
Sorry, there is not any employee with requested personal number. Do you want to repeat delete by entering the new personal number (y/n)? y
Please enter the personal number of an employee? 8921002
Do you really want to delete employee Michael Jackson (y/n)? y
The employee Michael Jackson has been deleted. Do you want to repeat delete by entering the new personal number (y/n)? n
3. UpdatePerson(…)
This function allows user to make changes in some fields of staff information. The field “Personal Number” is not allowed to be changed. For example, if user wants to change the number of working hours for specific employee, he should use this function. Since the only unique value in the list of employees is “Personal Number”, therefore update function should be based on this field. You could use the Figure 3.6 as a basic idea for your development. Note that during the update process, if user changes fields “working hours” or “salary of one hour work”, you need to re-calculate yearly salary based on new values and update related fields.
Please enter the personal number of an employee? 2425374
Sorry, there is not the employee with requested personal number. Do you want to repeat update by entering the new personal number (y/n)? y
Please enter the personal number of an employee? 8921001
Please enter the related number of field which you would like to update
(1. First name, 2. Family name, 3. Working hours per week, 4. Payment for one hour)? 4
Please enter new payment for one hour in Euros for Mr. Peterson? 25
Do you want to update any other field (y/n)? n
The information for Mr. Peterson has been updated. Do you want to repeat update by entering the new personal number (y/n)? n
4. ReportList(…)
In this function, user can choose a specific field to make reporting. These fields can be “Family Name” or “Salary”. You could use Figure 3.7 as a basic for your development. The list will be sorted ascending based on the requested field.
5. SearchPerson(…)
In this function, user can find specific people using chosen fields. These fields can be “Family Name” or “Salary”. You could use Figure 3.8 as a basic for your development.
By using salary as a search field, user should define minimum and maximum values for search. As a result he/she will get the list of employees who their salary is between requested Min_Salary and Max_salary. For searching the employee with specific amount of salary, user should define same Min_Salary and Max_Salary values.
By using the “Family Name” as a search field user will get the list of employees who their family name is equal to requested family name.
Search is based on two different fields (1.family name, 2.Salary), please enter your choice? 2
Please define your search range for salary of employees (S_min, S_max).
What is minimum salary for search (S_min)? 60000
What is maximum salary for search (S_max)? 110000
Following is the list of employees for your defined search fields:
First Name Family Name Personal Number salary (Euro)
————– —————- ———————- —————-
Martin Klein 8921003 81900.00
Sarah Müller 8921004 105300.00
Do you want to do new search (y/n)? y
Search is based on two different fields (1.family name, 2.Salary), please enter your choice? 1
Please enter the family name of employee? Peterson
Following is the list of employees for your defined search fields:
First Name Family Name Personal Number salary (Euro)
————– —————- ———————- —————-
Alex Peterson 8921001 45500.00
Do you want to do new search (y/n)? n
Solution-
#include<iostream> #include<math.h> #include<cstdlib> #include<string> using namespace std; class Person { private : string FirstName; // student name, max 49 characters string LastName; // student family name int PersonalID ; double Salary ; double WorkingHours ; double CostPerHour ; public : void set_FieldName(); void get_FieldName(); void gett_FieldName(); void set_PersonalID(); void set_Name(); void LastNamesortList(); void in_FirstName(); void in_FamilyName(); void in_Workinghour(); void in_Costperhour(); }; class HRM { private : Person e[100] ; Person temp[100]; public : void AddPerson(); void DeletePerson(); void SearchPerson(); void UpdatePerson(); void ReportList(); }; ////global variables declared int n=0,i=0,x=8248001,y[100]; bool flag=0; int z[100]; string h[100]; double sal[100]; int check=0; ///////////////////////////////////////////////////////////////////// // Definition of the AddPerson() method of the Company class // This method will add an employee to the Company class instance. // The attributes of the employee should be those of the user's // own choosing. void ::HRM::AddPerson() { int x; char redo; do { n++; e[i].set_FieldName(); i++; cout<<"\nThe employee with the following information has been added to the system:"<<endl; cout<<"\nFirst Name Last Name Personal ID Salary per year (Euros)"; cout<<"\n-------------- -------------- ------------ -------------------------"<<endl; //cout<<"naaaaaaaaaaaam"<< n<<endl; for(int i=0; i<n; i++){ e[i].get_FieldName(); } cout<<"do u wont to add another employee"<<endl; cin>>redo; } while((redo=='y'||redo=='Y')); } ///////////////////////////////////////////////////////////////////// // Definition of the DeletePerson() method of the Company class. // This method will delete the employee of the user's choosing from // the Company class instance. void HRM::DeletePerson() { // Show all of the current employees // Ask the user the ID of which employee that they wish to // delete. int empId; bool lol; char redo1,redo2; lol =false; lebel: cout << "ID of employee to remove: "; while(!(cin>>empId)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a number! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } for ( i = 0; i < n; ++i) { if (y[i]==empId) { e[i]=e[i+1]; lol=true; e[i].set_Name(); cin>>redo2; if(redo2=='Y'||redo2=='y'){ int a; a=n; cout<<"\nThe employee with the following information has been added to the system:"<<endl; cout<<"\nFirst Name Last Name Personal ID Salary per year (Euros)"; cout<<"\n-------------- -------------- ------------ -------------------------"<<endl; for(int i=0; i<a; i++){ e[i].get_FieldName(); cout<<"hahahahah="<<n<<endl; a--; n=a; n++; } /// goto lebel1; } cout <<endl; //Delete function } } if (lol==false) { cout<<"Sorry, there is not any employee with requested personal number." <<" Do you want to repeat delete by entering the new personal number (y/n)?:"; cin>>redo1; if(redo1=='Y'||redo1=='y'){ goto lebel; cout<<endl<<endl; } } // Delete the chosen employee from the array of employees // as represented in this class. // Actually remove the entry from memory so as to not leak objects //nextIndex--; } ///////////////////////////////////////////////////////////////////// // Definition of the UpdatePerson() method of the Company class // This method will modify an attribute of the employee in // the Company class instance. The employee and attribute should be // ones of the user's own choosing. void HRM::UpdatePerson(){ int empId; char redo1,redo2; lebel: cout << "ID of employee to modify data: "; while(!(cin>>empId)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a number! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } int flag1=0; for (int i = 0; i < n; ++i) { if (y[i]!=empId) { flag1++; } } /* if (flag1==n){ // cout<<" not matching="<< y[i]; cout<<"Sorry, there is not any employee with requested personal number. Do you want to repeat delete by entering the new personal number (y/n)?:"; cin>>redo1; if(redo1=='Y'||redo1=='y'){ goto lebel; } } */ cout <<endl; lebel1: for (int i = 0; i < n; ++i) { if (y[i]==empId) { cout<<"matching="<< y[i]; flag=true; int choice = 0; char redo; do { cout << endl << endl; cout << "Please enter the related number of field which you would like to update" << endl; cout <<"1. First name" << endl; cout << "2. Family name" << endl; cout << "3. Working hours per week" << endl; cout << "4. Payment for one hour" << endl; cout << std::endl; cin >> choice; if (choice == 1) { cout << " First name: "; e[i].in_FirstName(); } else if (choice == 2) { cout << " Family name: "; e[i].in_FamilyName(); } else if (choice == 3) { cout << " Working hours per week: "; e[i].in_Workinghour(); } else if (choice == 4) { cout << " Payment for one hour: "; e[i].in_Costperhour(); } cout<<"Do you want to update another field (Y/N)="; cin>>redo; } while (redo=='y'||redo=='Y'); } } int a; a=n; cout<<"\nThe employee with the following information has been added to the system:"<<endl; cout<<"\nFirst Name Last Name Personal ID Salary per year (Euros)"; cout<<"\n-------------- -------------- ------------ -------------------------"<<endl; for(int i=0; i<a; i++){ e[i].get_FieldName(); cout<<"hahahahah="<<n<<endl; } } void ::Person::in_FirstName() { cin>>FirstName ; } void::Person::in_FamilyName() { cin>>LastName; } void::Person::in_Workinghour() { cin>>WorkingHours; Salary=WorkingHours*CostPerHour*52; } void::Person::in_Costperhour() { cin>>CostPerHour; Salary=WorkingHours*CostPerHour*52; } void HRM::ReportList() { char op; bool doMore; cout<<"\nPlease enter the related number of the field which you would like to sort the list based on it.\n(1. Family name, 2.Salary)?\n"<<endl; //cin>>op; while(!(cin>>op)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a number! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } switch(op) { case '1': cout<<"\nSorting based on Family Name\n"<<endl; for(int i=0;i<=n;i++) { for(int j=i+1;j<=n-1;j++) { if(h[i]>h[i+1]) { temp[i]=e[i]; e[i]=e[j]; e[j]=temp[i]; } } } for(int i=0;i<n;i++) { e[i].get_FieldName(); } cout<<"\nsorted\n"; break; case'2': cout<<"\nSorting based on Salary\n"<<endl; for(int h=0;h<n;h++) { for(int q=h+1;q<n;q++) { if(sal[h]>sal[h+1]); { temp[h]=e[h]; e[h]=e[q]; e[q]=temp[h]; } } } for(int j=0;j<n;j++) { e[j].get_FieldName(); } cout<<"\nsortedlist is printed above\n"; break; } } void HRM::SearchPerson(){ int c; char redo1; string familyname; double min,max; do{ cout<<"Search is based on two different fields (1.family name, 2.Salary), please enter your choice?="<<endl; //cin>>c; while(!(cin>>c)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a number! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } if(c==2) { cout<<"Please define your search range for salary of employees ."<<endl; cout<<"What is minimum salary for search (S_min)?="<<endl; //cin>>min; while(!(cin>>min)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a number! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } cout<<"What is maximum salary for search (S_max)?="<<endl; //cin>>max; while(!(cin>>max)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a number! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } int a; a=n; cout<<"\nThe employee with the following information has been added to the system:"<<endl; cout<<"\nFirst Name Last Name Personal ID Salary per year (Euros)"; cout<<"\n-------------- -------------- ------------ -------------------------"<<endl; for(int i=0; i<n; i++) { if (z[i]>min && z[i]<max) { cout<<"naaaaaaaaaaaam"<< n<<endl; e[i].gett_FieldName(); cout<<"matching="<< z[i]; } } } else if(c==1) { cout<<"Please enter the family name of employee?"<<endl; cin>>familyname; cout<<"\nThe employee with the following information has been added to the system:"<<endl; cout<<"\nFirst Name Last Name Personal ID Salary per year (Euros)"; cout<<"\n-------------- -------------- ------------ -------------------------"<<endl; for(int i=0; i<n; i++) { if (h[i]==familyname) { cout<<"naaaaaaaaaaaam"<< n<<endl; e[i].gett_FieldName(); cout<<"matching="<< z[i]; } } } cout<<"\nDo you want to Search any other field (y/n)?\n"<<endl; cin>>redo1; }while(redo1=='y'||redo1=='Y'); } void ::Person::LastNamesortList() { int temp2; char temp,temp1; } void::Person ::set_Name(){ cout<<"Do you really want to delete employee"<<FirstName<<"\t"<< LastName<< "(y/n)?:"; //PersonalID=y[i]; } int k=0; void::Person ::set_FieldName(){ PersonalID=x; x++; cout<<n; cout<<"First Name="; while(!(cin>>FirstName)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a string! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } cout<<"Last Name="; while(!(cin>>LastName)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a string! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } cout<<"How many hours a week is his/her work? ="; ; while(!(cin>>WorkingHours)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a number! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } cout<<"How much per hour is his/her salary? ="; while(!(cin>>CostPerHour)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a number! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } Salary=WorkingHours*CostPerHour*52; z[i]= Salary; sal[i]=Salary; h[i]=LastName; y[k]=PersonalID; k++; } void::Person ::get_FieldName(){ cout<<FirstName<<"\t\t"<<LastName<<"\t\t\t"<<PersonalID<<"\t\t\t"<<Salary<<"\t"<<endl; } void::Person ::gett_FieldName(){ cout<<FirstName<<"\t\t"<<LastName<<"\t\t\t"<<PersonalID<<"\t\t\t"<<Salary<<"\t"<<endl; } int main() { //-------defining variables and initializing them------------- HRM info ; int c; char operation,ch; //--------Printing my name on screen---------------- cout<< "Welcome to the program 2.1 written by Your Name"<<endl; cout<<"**************************************************************************"<<endl; cout<<endl<<endl<<endl; do { cout<<"Welcome to Human Resource Management (HRM) Software of Company XYZ."; cout<<"To do specific task please choose one of the following commands."<<endl<<endl<<endl; cout<<" 1. Add new employee"<<endl; cout<<" 2. Delete employee information"<<endl; cout<<" 3. Update employee information"<<endl; cout<<" 4. Make reports based on specific field"<<endl; cout<<" 5. Search employee"<<endl; cout<<" 6. Quit"<<endl<<endl; while(!(cin>>c)) //Reciving vaiables from input : is it no/character ? { cout << "Please enter a number! Try again: "; cin.clear (); cin.ignore (1000, '\n'); // Skip to next newline or 1000 chars, // whichever comes first. } switch(c) { case 1: cout<<"\nEnter the information of the new employee"<<endl; info.AddPerson(); break; case 2: info.DeletePerson(); break; case 3: cout<<"\nEnter an item to deletion"; info.UpdatePerson(); break; case 4: cout<<"\nEnter an element to search"; info.ReportList(); break; case 5: info.SearchPerson(); break; default : cout<<"\nInvalid option try again"; } cout<<"\ndo u want to continue"; cin>>ch; } while(ch=='y'||ch=='Y'); system("pause"); return 0; }
- C++ Simple Programs And Examples
- C++ – Hello World Program
- C++ – Simple calculator
- C++ – Even and Odd
- C++ – Swap two numbers
- C++ – Prime Number
- C++ – Find Perfect Number
- C++ – Factorial of Number
- C++ – Fibonacci Series
- C++ – Human Resource Management Program
- C++ – Calculate number of characters, words, sentences
- C++ – Subtract two Strings
- C++ – Processing of the Students structure
- C++ – Program with Matrices
- C++ – Calculate Equation
- C++ – Arrange Numbers in Ascending order
- C++ – Check Armstrong Number
- C++ – HCF and LCM
- C++ – Square Root of a Number
- C++ – Cube Root of a Number
- C++ – ASCII Code for Characters and numbers
- C++ – Check Palindrome Number
- C++ – Bubble sort
- C++ – Random Number Generator
- C++ – Sum of ODD Numbers in the Given Range
- C++ – Display current date and time
- Formula Based Programs
- C++ – Leap Year
- C++ – Surface Area and volume of cone
- C++ – Solve Quadratic equation
- String Based Programs
- C++ – String into Upper case or lower case
- C++ – Concatenate Strings
- Array Based Programs
- C++ – Program with Matrices
- Print Any Patterns
- C++ – Print 5 rows of 10 Stars (*)
- C++ – Half Pyramid of Stars (*)
- C++ – Half Pyramid of numbers
- C++ – Print Triangle of Stars
- C++ – Display Reverse pyramid.
- C++ – Print Alphabet Pattern
- C++ – Diamond of Star
- C++ – Pascal Triangle
- C++ – Floyd Triangle
- C++ Conversion
- C++ – Convert decimal to Hexadecimal
- C++ – Decimal to Binary
- C++ Sorting algorithms & Techniques
- C++ – Bubble Sort
- C++ – Insertion Sort
- C++ – Selection Sort
- C++ – Merge Sort
- C++ – Quick Sort
- C++ Handling Files
- C++ – How to get Current Directory in Linux/Windows
- C++ – How Create a Text File and Write in It
Leave a Reply