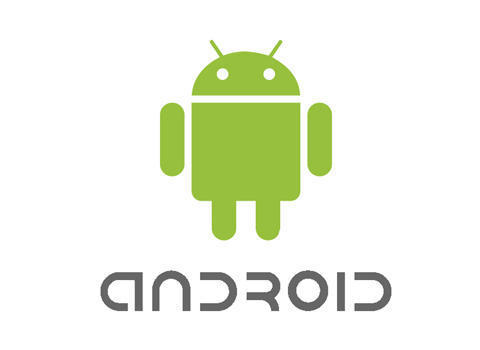
package com.example.programmingknowledge.mysqldemo; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.EditText; import android.widget.Toast; public class MainActivity extends ActionBarActivity { EditText UsernameEt, PasswordEt; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); UsernameEt = (EditText)findViewById(R.id.etUserName); PasswordEt = (EditText)findViewById(R.id.etPassword); } public void OnLogin(View view) { String username = UsernameEt.getText().toString(); String password = PasswordEt.getText().toString(); String type = "login"; BackgroundWorker backgroundWorker = new BackgroundWorker(this); backgroundWorker.execute(type, username, password); } }
package com.example.programmingknowledge.mysqldemo; import android.app.AlertDialog; import android.content.Context; import android.os.AsyncTask; import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.io.OutputStream; import java.io.OutputStreamWriter; import java.net.HttpURLConnection; import java.net.MalformedURLException; import java.net.URL; import java.net.URLEncoder; /** * Created by ProgrammingKnowledge on 1/5/2016. */ public class BackgroundWorker extends AsyncTask<String,Void,String> { Context context; AlertDialog alertDialog; BackgroundWorker (Context ctx) { context = ctx; } @Override protected String doInBackground(String... params) { String type = params[0]; String login_url = "http://192.168.1.6/login.php"; if(type.equals("login")) { try { String user_name = params[1]; String password = params[2]; URL url = new URL(login_url); HttpURLConnection httpURLConnection = (HttpURLConnection)url.openConnection(); httpURLConnection.setRequestMethod("POST"); httpURLConnection.setDoOutput(true); httpURLConnection.setDoInput(true); OutputStream outputStream = httpURLConnection.getOutputStream(); BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(outputStream, "UTF-8")); String post_data = URLEncoder.encode("user_name","UTF-8")+"="+URLEncoder.encode(user_name,"UTF-8")+"&" +URLEncoder.encode("password","UTF-8")+"="+URLEncoder.encode(password,"UTF-8"); bufferedWriter.write(post_data); bufferedWriter.flush(); bufferedWriter.close(); outputStream.close(); InputStream inputStream = httpURLConnection.getInputStream(); BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream,"iso-8859-1")); String result=""; String line=""; while((line = bufferedReader.readLine())!= null) { result += line; } bufferedReader.close(); inputStream.close(); httpURLConnection.disconnect(); return result; } catch (MalformedURLException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } return null; } @Override protected void onPreExecute() { alertDialog = new AlertDialog.Builder(context).create(); alertDialog.setTitle("Login Status"); } @Override protected void onPostExecute(String result) { alertDialog.setMessage(result); alertDialog.show(); } @Override protected void onProgressUpdate(Void... values) { super.onProgressUpdate(values); } }
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" android:id="@+id/myrl"> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="textPersonName" android:ems="10" android:id="@+id/etUserName" android:layout_alignParentTop="true" android:layout_marginTop="47dp" /> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="textPassword" android:ems="10" android:id="@+id/etPassword" android:layout_below="@+id/etUserName" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Login" android:id="@+id/btnLogin" android:layout_below="@+id/etPassword" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_marginLeft="48dp" android:layout_marginStart="48dp" android:layout_marginTop="50dp" android:onClick="OnLogin"/> </RelativeLayout>
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.programmingknowledge.mysqldemo" > <uses-permission android:name="android.permission.INTERNET"></uses-permission>" <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
<?php $db_name = "employee101"; $mysql_username = "root"; $mysql_password = ""; $server_name = "localhost"; $conn = mysqli_connect($server_name, $mysql_username, $mysql_password,$db_name); ?>
<?php require "conn.php"; $user_name = $_POST["user_name"]; $user_pass = $_POST["password"]; $mysql_qry = "select * from employee_data where username like '$user_name' and password like '$user_pass';"; $result = mysqli_query($conn ,$mysql_qry); if(mysqli_num_rows($result) > 0) { echo "login success !!!!! Welcome user"; } else { echo "login not success"; } ?>
Video Instructions
Hey Prabeesh
I have used your codes to learn. Just the way it is . The program installand when I try to run ,The screen is stuck with ‘Login Status’
Sorry got it working.
please i have the same error When i click the LOgin Button the AlertDialog is empty!! there is only the title that i set in onPreExecute()..and throw IO Exception . Any ideas about my problem?
it is worked .. my manifest.xml had an error ..it shoud be like that
not like that
what u fixed in manifest.xml file?
How did you fix it?
nice
Hello, I just watched your “Android MySQL Database Tutorial – Android Login with PHP MySQL” video on youtube. it’s awesome.
bt problem is i have done like yours bt when i click on login button it shows alert box bt not successful msg
You can use the android studio virtual device then may be it will work.
Actually i m also using genymotion but in that the same code is not working.
but when i thought to run with android own vitual device then it is working fine so do that may be it will work for u too as it is working for me.
I have same problem ! did you find any solution?
Same problem need help
please i have the same error When i click the LOgin Button the AlertDialog is empty!! there is only the title that i set in onPreExecute()..and throw IO Exception . Any ideas about my problem?
Hello,
Very useful code. Thank you for that! I have a problem though and I cannot manage to find the reason. As I have a hosting account I did this tutorial directly on a sql data base that I created online. My hosting is with Ipage. They have a Cpanel but is not exactly how you show it in your video. I have followed every step created everything without any error but now when I launch the app on my phone and I introduce the username and password the answer is always the Alert dialog (Login Status) and the message from login.php login not success. Where do you think could be the problem? Once I get the message from the login.php it means that the app connects to the online files but somewhere here there is a problem $result = mysqli_query($conn ,$mysql_qry);
if(mysqli_num_rows($result) > 0)
Please any suggestion is welcomed!
try this line in your login.php file:
$mysql_qry = “select * from employee_data where user_name ='”.$user_name.”‘ and password='”.$user_pass.”‘”;
Hello i have Also the Same problem. please if you solve the problem then please reply me.
In my case the problem was that in the php file I had an extra space. So check it propperly as a single extra space can mess up the result of the connection.
but how can i find the extra space .can you send me snapshot on my email id sahrishkhan534@gmail.com
thanks a lot my problem is solve .this is best method
Hey there,
I have the same problem as you, but in my case, I have no idea where I can get an extra space in php file… Any other suggestions?
Thanks in advance.
send me the pictures of php files i will highlight the spaces.
Here you are and thanks a lot! https://uploads.disquscdn.com/images/89a5f6a576f91d4c8283487253136885333c816477f45f70fc82abaf8b96b1bc.png
0){
echo “login success “;
}
else {
echo “login not success”;
}
?>
your query was wrong
your database name is lekarz?
yes, I’ve created slightly different database to my thesis. Thanks a milion! You saved my life – literally 🙂
you can mail me your all coding of php on my email id sahrishkhankhan@gmail.com..i try to solve
hey bro r u solve this myproblem?
what is is your problem ? mention it.
$mysql_qry = “select * from employee_data where user_name ='”.$user_name.”‘ and password='”.$user_pass.”‘”;
$result = mysql_query($mysql_qry) ;
if(mysql_num_rows($result) > 0) {
echo “login success !!!!! testeeooo”;
}
else {
echo “login not success”;
}
in these code it will give login not sucess on app application but it will run on cpanel or browser
0){
echo “login success !!!!! testeeooo”;
}
else {
echo “login not success”;
}
?>
try that code because in your code you missed specific word like.
bro after click on login button it will show alert box with connection success……..but login unsuccessfull
0){
echo “login success !!!!! testeeooo”;
}
else {
echo “login not success”;
}
?>
hey bro can you solve this problem? because i face same problem
with the same code i have a error
javax.net.ssl.SSLHandshakeException: Handshake failed
Caused by: javax.net.ssl.SSLProtocolException: SSL handshake aborted: ssl=0xad51f980: Failure in SSL library, usually a protocol error
error:100bd10c:SSL routines:ssl3_get_record:WRONG_VERSION_NUMBER
any ideas?
Very very good. Everything it’s fine..The only parts I had to change were in php (maybe a version issue):
1- conn.php file
This works fine:
$link = mysql_connect($host, $username, $password);
if (!$link) {
die(‘Error al conectarse a mysql: ‘ . mysql_error());
}
$db_selected = mysql_select_db($database, $link);
2- login.php file
$mysql_qry = “select * from employee_data where user_name ='”.$user_name.”‘ and password='”.$user_pass.”‘”;
$result = mysql_query($mysql_qry) ;
if(mysql_num_rows($result) > 0) {
echo “login success !!!!! testeeooo”;
}
else {
echo “login not success”;
}
Hi, thanks for the great tutorial,
Hopefully this may help you all. I had the same issue with the Login dialog. Here is what worked for me. In this line below, I did not notice the semi colon between the single quotation mark and the last double quotation marks in the video. I added the semi colon and now it is giving the successful message and working fine.
$mysql_qry = “select * from employee_data where username like ‘$user_name’ and password like ‘$user_pass’;”;
Hope this can be of help.
Hello Guys am Facing some type of problem can anyone help me out!? https://uploads.disquscdn.com/images/5b5ce22fd834358944fe4a1150b99941640604ddcbcc67ac50aa5779750af9ce.png
Hi! 1st of all thanks a lot for your, all very very useful tutorials… i just got a problem here is… whenever i am clicking on login button it’s just showing “Login Status” title but not showing any message like login success or not success.
I face same problem, please help us
did you find the solution sir?
please help..!!
http://disq.us/p/1lc5dtl
when i login than i get this error
“0) { echo “login success !!!!! Welcome user”; } else { echo “login not success”; } ?>”
did you find the solution ma’am?
please help..!!
did you find the solution sir?
please share the solution..!!
Yes there was just a syntax error in my script… the script is correct, you just need to check it properly… maybe there’s a syntax error.
i’m facing the same problem. Could you please help me
How to add progressbar and get flag(from php) success and fail login to Process on android, thanks
For progressbar follow following 3 steps.
—>//Declare object of ProgressDialog in BackgroundWorker.Do not forget to import android.app.ProgressDialog class.
ProgressDialog pd1 ;
—>//Add this code in onPreExecute method.
pd1 = new ProgressDialog(context);
pd1.setTitle(“Retrieving data”);
pd1.setMessage(“Please wait.”);
pd1.setCancelable(true);
pd1.setIndeterminate(true);
pd1.show();
—> //Add this code in onPostExecute method.
if(pd1!=null) pd1.dismiss();
when login button is clicked alertDialog says LOGIN STATUS…
when register button is pressed from register activity alertDialog again says LOGIN STATUS…..how to change it to display as “REGISTER STATUS”…?
Hello, Can you please tell me how to open new activity if login is successed! I tried startActivity method it is giving error that can n’t resolve the constructor Intent in current class is less than new activity. Please help me.
Use
Context instead of this nd
Context.startActivity(in);
i have same problem
can anyone please help?
Excuse me,have you solved this problem? I need help please QQQ
Thanks 😀
hi how can i retrieve data from database and display it on the table
Use jsonparser
will you please give me a code for retrieve data and display it
plz hlp me.
guys help me my connection is successful but when I login in it it shows my data it shows only html code please help me guys how html embeeded and it shows data.
when i run the application on the AVD, it asks for the username and password , but when i click on the login, the application closes down. any idea?
i am using online database not a local
try giving access permission for your IP in remote server
what are the dependencies you have added sir? im not able to get OnPreExecute method. pls resopnd ASAP
When I click login button, it shows login status only, why?
Same problem 🙁
me too
the problem in this line > String login_url = “http://192.168.1.6/login.php”;
edit it to >> String log_url = “http://10.0.2.2/webapp/login.php”;
ATTENTION : edit this webapp to your folder name where login.php exist
if the probelm still , then ensure your folder name is right
SOLVED
the problem in this line > String login_url = “http://192.168.1.6/login.php”;
edit it to >> String login_url = “http://10.0.2.2/webapp/login.php”;
ATTENTION : edit this webapp to your folder name where login.php exist
if the probelm still , then ensure your folder name is right !
if you use XAMPP like me , then it will be >> String login_url = “http://10.0.2.2:81/login.php”;
ATTENTION : instead of 81 use your port number
still problem exist
only login status comes
are you using phone or emulator?
that problem is solved thanku for reply
one thing i have to ask if i want to connect with jdbc then how i can host in and which hosting site is prefered
guys help me my connection is successful but when I login in it it shows my data it shows only html code please help me guys how html embeeded and it shows data. plz help me
guys help me my connection is successful but when I login in it it shows my data it shows only html code please help me guys how html embeeded and it shows data
@Pearl Adams hey I m using xamp too and i have same problem but in your solution u said use port no but i want to run on real device in my own mobile so wht should I do please help me
you have to edit like :
String login_url = “http://192.168.1.6/webapp/login.php?”;
you have add question mark after php file.
In xampp there will be a htdocs folder
Place your php in htdocs folder(php will basically fetch and set data in database….server)
Open command prompt and type ipconfig.
There infront of ipv4 you will find an address like 192.xx.xx.xx
Copy that address and save it somewhere. Remember ip will change whenever you’ll change wifi or reset it or restart your system so you’ll have to build app again if you restart sytem, etc.
Add this line in your android manifest
android:usesCleartextTraffic=”true”
how to open new activity when login succeed??
In onPostExecute() – Intent intent = new Intent(yourActivity.this,anotherActivity.class);
startActivity(intent);
hey Марио Герасимов man how can I use this line check that picture how to res https://uploads.disquscdn.com/images/b0f93285e612ab52be089e90bee89c7e6a3d0c61c94510fa5c6de96559d37051.png olve thei symbol error like in another Activity and start activity
give some error
Excuse me,have you solved this problem? I need help please QQ
if(ed1.getText().toString().equals(“admin”) && ed2.getText().toString().equals(“admin”)) {
Toast.makeText(getApplicationContext(),”Login Successfull.”,Toast.LENGTH_SHORT).show();
Intent intent = new Intent(LoginActivity.this, LanguagesActivity.class);
intent.putExtra(“username”, ed1.getText().toString());
startActivity(intent);
// Intent i = new Intent(CurrentActivity.this, SecondActivity.class);
//startActivity(i);
Did you solve this problem? How did it work?
how to move into new activity after login success ? the OnPreExecute need to change ?
I really thank you bro for your helpful tutorials. I’ve been studying programming from you since four years. Many thanks and best wishes for you brother.
don’t work , only show (login stus), I falow the code but!!
so the code works perfectly on an emulator. but when i try on my phone it only shows login status and the if it was success or not. please help. i even put my ip address and i get the same results on both devices.
guys help me my connection is successful but when I login in it it shows my data it shows only html code please help me guys how html embeeded and it shows data.
hi. i keep getting the login status without calling the php file. can i know why? and how to solve it?
After successful login i want to redirect it to a new page . for that what should i do.
i am using a webhosting server and when i run the app on my phone i get the box login status ..
But no more, is there anyone who has any suggestions?
I have changed String login_url to the right one
Hello guys
Please, i don’t find easyonlineconverter.com
Someone have a link ?
or another link of website
thanks
Hey Guys can you help me ? how to Login Admin and User in different Activity ?
Hello , I am new:
I want; whenever user gets ‘login success’ the user will get alert with Ok …and when this Ok is clicked then user should go to Activity2 Page.
I am unable to do this please help; below is the code:
@Override
protected void onPostExecute(String result) {
alertDialog.setMessage(result);
if(result.equals(“login success”)) {
alertDialog.setButton(“OK”, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
setContentView(R.layout.activity_main2);
}
});
}
else {
}
alertDialog.show();
}
Hello guys…. I’m new in android programming but I’m conversant with php…. the above code is working very fine with me, now plz how do I handle my session and navigate to profile page showing the users his/her name in inside the button…. Thanks Admin,Friends and all programmers in this forum…
why i go to next activity
hello i have an issues where the variables username and password are showing undefined when using post method index when i launch the login.php file on the browser so unable to proceed further so could you please help me!!!!.
How can i open a new activity when i click on the button “login” when the password is correct