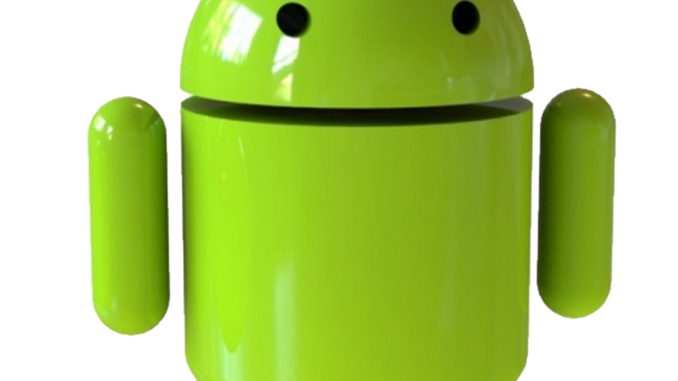
Like internal storage, we can spare or read information from the gadget outer memory, for example, sdcard. FileInputStream and FileOutputStream classes are utilized to peruse and compose information into the document.
Case of perusing and composing information in the android outside capacity…
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" > <EditText android:id="@+id/editText1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_alignParentTop="true" android:layout_marginRight="20dp" android:layout_marginTop="24dp" android:ems="10" > <requestFocus /> </EditText> <EditText android:id="@+id/editText2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignRight="@+id/editText1" android:layout_below="@+id/editText1" android:layout_marginTop="24dp" android:ems="10" /> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBaseline="@+id/editText1" android:layout_alignBottom="@+id/editText1" android:layout_alignParentLeft="true" android:text="File Name:" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBaseline="@+id/editText2" android:layout_alignBottom="@+id/editText2" android:layout_alignParentLeft="true" android:text="Data:" /> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/editText2" android:layout_below="@+id/editText2" android:layout_marginLeft="70dp" android:layout_marginTop="16dp" android:text="save" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBaseline="@+id/button1" android:layout_alignBottom="@+id/button1" android:layout_toRightOf="@+id/button1" android:text="read" /> </RelativeLayout>
You need to provide the WRITE_EXTERNAL_STORAGE permission.
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
Activity_Manifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.externalstorage" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="16" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.example.externalstorage.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
MainActivity.java
package com.codebind.externalstorage; import java.io.BufferedReader; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStreamReader; import java.io.OutputStreamWriter; import android.os.Bundle; import android.app.Activity; import android.content.Context; import android.view.Menu; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; public class MainActivity extends Activity { EditText editTextFileName,editTextData; Button saveButton,readButton; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); editTextFileName=(EditText)findViewById(R.id.editText1); editTextData=(EditText)findViewById(R.id.editText2); saveButton=(Button)findViewById(R.id.button1); readButton=(Button)findViewById(R.id.button2); //Performing action on save button saveButton.setOnClickListener(new OnClickListener(){ @Override public void onClick(View arg0) { String filename=editTextFileName.getText().toString(); String data=editTextData.getText().toString(); FileOutputStream fos; try { File myFile = new File("/sdcard/"+filename); myFile.createNewFile(); FileOutputStream fOut = new FileOutputStream(myFile); OutputStreamWriter myOutWriter = new OutputStreamWriter(fOut); myOutWriter.append(data); myOutWriter.close(); fOut.close(); Toast.makeText(getApplicationContext(),filename + " saved",Toast.LENGTH_LONG).show(); } catch (FileNotFoundException e) {e.printStackTrace();} catch (IOException e) {e.printStackTrace();} } }); //Performing action on Read Button readButton.setOnClickListener(new OnClickListener(){ @Override public void onClick(View arg0) { String filename=editTextFileName.getText().toString(); StringBuffer stringBuffer = new StringBuffer(); String aDataRow = ""; String aBuffer = ""; try { File myFile = new File("/sdcard/"+filename); FileInputStream fIn = new FileInputStream(myFile); BufferedReader myReader = new BufferedReader( new InputStreamReader(fIn)); while ((aDataRow = myReader.readLine()) != null) { aBuffer += aDataRow + "\n"; } myReader.close(); } catch (IOException e) { e.printStackTrace(); } Toast.makeText(getApplicationContext (),aBuffer,Toast.LENGTH_LONG).show(); } }); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.activity_main, menu); return true; } }
Android Books To Learn Mobile Apps Programming
- Android Programming: The Big Nerd Ranch Guide
- Android Design Patterns: Interaction Design Solutions for Developers
- Android Application Development Cookbook – Second Edition
- Android User Interface Design: Turning Ideas and Sketches into Beautifully Designed Apps (Usability)
- Android Recipes: A Problem-Solution Approach for Android 5.0
- Hello, Android: Introducing Google’s Mobile Development Platform (Pragmatic Programmers)
- Beginning Android Games
Leave a Reply